CSS margin, padding, box-sizing, border
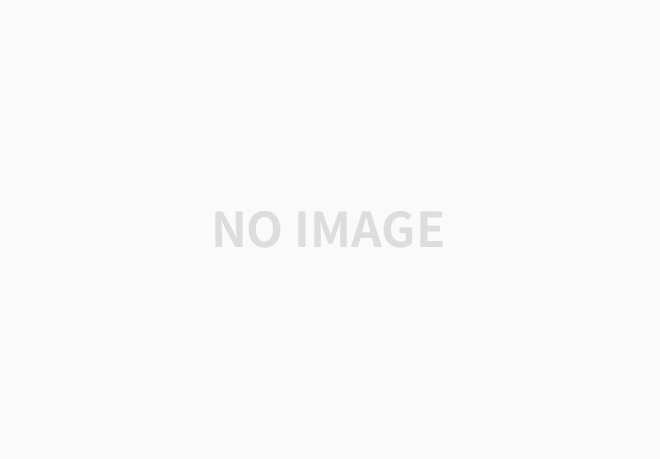
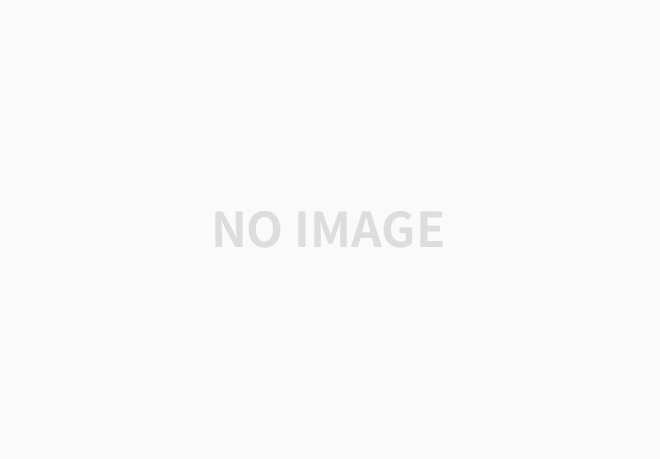
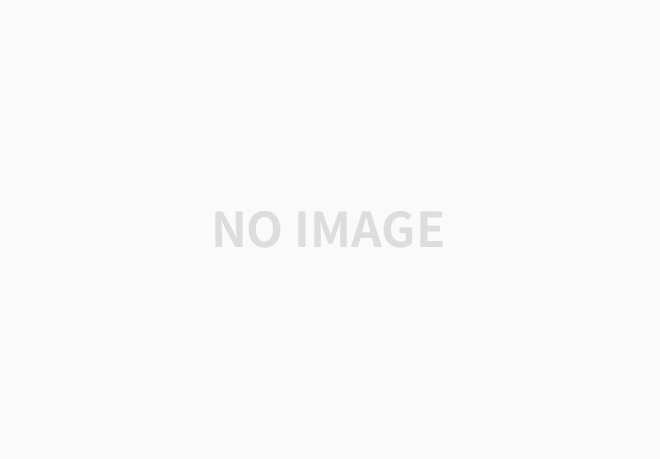
1. margin
- 테두리를 기준으로 바깥여백 지정
- 기본적으로 body 태그에는 8px 마진이 존재
- 마진에는 중첩 현상이 일어남 (같은 영역에 마진이 적용되면, 그 중 큰 마진으로 적용될 뿐 누적되지는 않음)
margin : 10px; | 모든 방향에 적용 |
margin : 10px 10px; | top,bottom / right,left 적용 |
margin : 10px 10px 10px; | top / right,left / bottom 적용 |
margin : 10px 10px 10px 10px; | top / right / left / bottom 적용 |
margin : auto; | 웹페이지 가운데 위치 |
margin-top | 위쪽 바깥 여백 |
margin-right | 오른쪽 바깥 여백 |
margin-bottom | 아래쪽 바깥 여백 |
margin-left | 왼쪽 바깥 여백 |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body{
margin: 0px
}
#box{
width: 100px;
height: 100px;
background-color: aquamarine;
margin : auto;
}
#box1,#box2,#box3,#box4{
width: 100px;
height: 100px;
}
#box1{
background-color: lightblue;
}
#box2{
background-color: lightcoral;
margin-left: 100px;
}
#box3{
background-color: lightgoldenrodyellow;
margin-left: 200px;
}
#box4{
background-color: lightsteelblue;
margin-left: 300px;
}
</style>
</head>
<body>
<h3>예제</h3>
<div id="box">내용</div>
<h3>실습</h3>
<div id="box1"></div>
<div id="box2"></div>
<div id="box3"></div>
<div id="box4"></div>
</body>
</html>
예제
내용
실습
2. padding
- 테두리를 기준으로 안쪽 여백을 지정하는 속성
- 테두리와 내용 사이의 여백
- 크기가 커진 것처럼 보일 수 있음
※ 적용 방법은 margin과 같음
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#box{
width: 100px;
height: 100px;
background-color: yellow;
padding-left: 30px;
padding-top: 20px;
}
#box1,#box2,#box3,#box4{
width: 100px;
height: 100px;
}
#box1{
background-color: lightblue;
}
#box2{
background-color: lightcoral;
padding-left: 100px;
}
#box3{
background-color: lightgoldenrodyellow;
padding-left: 200px;
}
#box4{
background-color: lightsteelblue;
padding-left: 300px;
}
</style>
</head>
<body>
<h3>예제</h3>
<div id="box">내용</div>
<h3>실습</h3>
<div id="box1">내용</div>
<div id="box2">내용</div>
<div id="box3">내용</div>
<div id="box4">내용</div>
</body>
</html>

3. box-sizing
- 요소의 크기를 화면에 표시하는 방식
① content-box (기본값)
내가 설정한 너비 = 컨텐츠의 너비
컨텐츠의 영역만을 크기로 삼는 속성
패딩, 보더 값이 들어가면 들어간 만큼 크기가 커진다
② border-box
내가 설정한 너비 = border 안쪽까지의 너비
content + padding + border = 내가 설정한 너비
패딩, 보더 값이 들어가도 크기가 커지지 않고 컨텐츠가 작아진다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
/* 내가 설정한 너비 */
width: 500px;
/* 경계선 */
border: 20px solid gray;
/* 안쪽 여백 */
padding: 20px;
}
.bb{
box-sizing: border-box;
}
</style>
</head>
<body>
<div class="cb">
<p>content-box</p>
</div>
<div class="bb">
<p>border-box</p>
</div>
</body>
</html>



4. border
- 요소의 테두리
- 선 두께, 선 종류, 선 색깔, ...
- border-style, border-width(테두리 두께), border-color(테두리 색깔), border-radius(테두리 모서리를 둥글게 만들기)

border-radius : 경계선을 둥글게 만들어 주는 속성
border-위/아래-좌/우-radius
border radius
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 100px;
height: 100px;
background-color: green;
border-top-left-radius: 40px;
border-bottom-right-radius: 40px;
}
</style>
</head>
<body>
<div></div>
</body>
</html>

▶ 실습 : 신호등 만들기
검정색 배경 : width 120px, height 360px
삼색 신호등 : width 100px, height 100px
단, 빨간색 원에 margin 주지 않기

코드 열기
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
/* 신호등 검정색부분 box1,box2,box3 */
#box1,#box2,#box3{
width: 120px;
height: 120px;
background-color: black;
padding-left: 10px;
padding-right: 10px;
box-sizing: border-box;
}
/* 신호등 빨강색, 노랑색, 초록색 */
#box1>div,#box2>div,#box3>div{
width: 100px;
height: 100px;
border-radius: 100px;
}
#box1{
padding-top: 20px;
border-top-left-radius: 20px;
border-top-right-radius: 20px;
}
#box2{
padding-top: 10px;
}
#box3{
border-bottom-left-radius: 20px;
border-bottom-right-radius: 20px;
}
#box1>div{
background-color: red;
}
#box2>div{
background-color: yellow;
}
#box3>div{
background-color: green;
}
</style>
</head>
<body>
<div id="box1">
<div></div>
</div>
<div id="box2">
<div></div>
</div>
<div id="box3">
<div></div>
</div>
</body>
</html>
'HTML CSS JS' 카테고리의 다른 글
CSS Position (0) | 2023.01.10 |
---|---|
CSS Flex (0) | 2023.01.09 |
CSS display (0) | 2023.01.08 |
CSS 선택자 (0) | 2023.01.05 |
CSS 기본, 폰트(font) (0) | 2023.01.05 |
댓글